TL;DR: Do you want to transform algebraic expressions into a series of operations for a mechanical calculator? Click here. Then come back and read the rest.
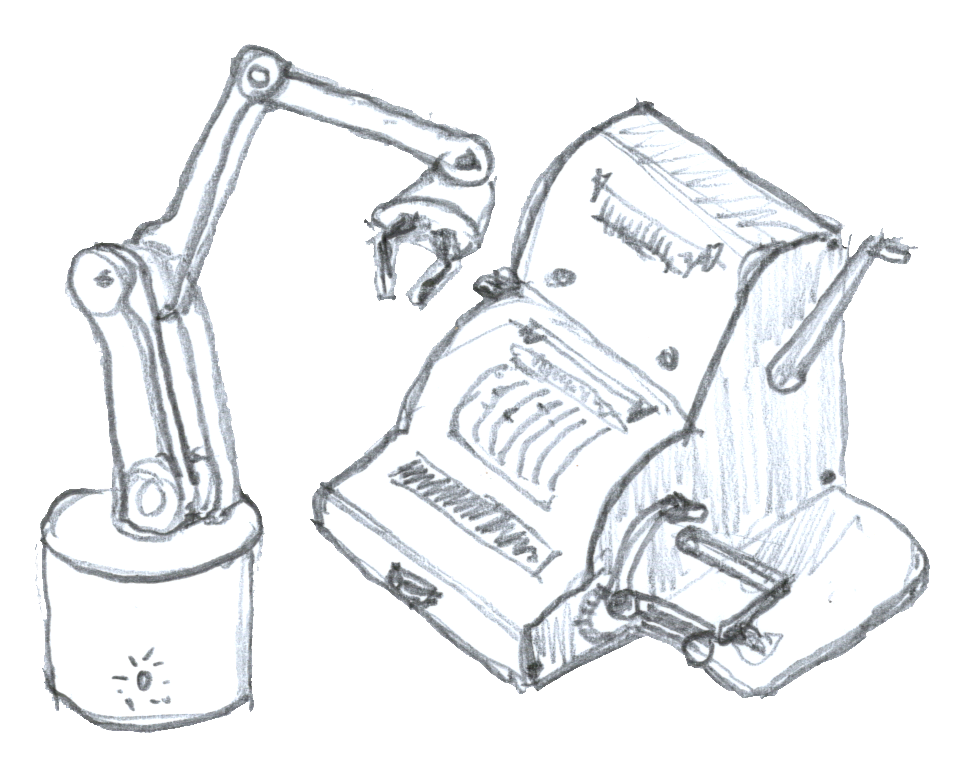
How about a web-enabled mechanical calculator?
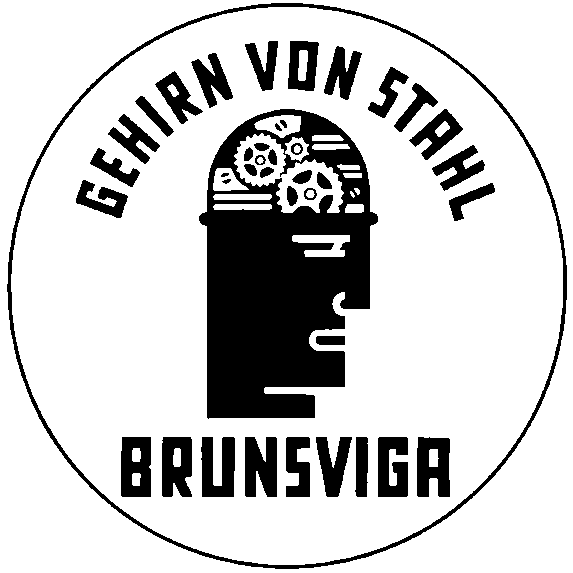
This article describes vapourware. It is about a project that currently only exists in my head. Every few months it tries to escape into the physical world. So far I have managed to wrestle it back every time after merely wasting a few hours of web research.
The Machine
I have been given a mechanical calculator as a present:
This is a Brunsviga 13RK. It can perform addition, subtraction, multiplication and division. These machines were mass-produced between 1952 and 1964. They are still quite common and have a nice workhorse-flair which is not the least conveyed by their hefty weight of about 8 kilos.
When I got it I downloaded the manual for it and rehearsed calculations to demonstrate to myself (and anyone who did not run away screaming) how this used to work. I have even divided by zero on it to make a point.
Then I relegated it to my shelf of Nice Stuff That is Not Useful Anymore.
But…
The Vision
At the back of my head a little voice is nagging me to web-enable this purely mechanical device that was built before electronic calculators became commonplace, let alone general-purpose computers.
I would love to make a robotic arm operate it. it would be controlled by a small stand-alone computer connected to the internet. The controller would export a web interface where users around the world could enter any expression that uses the operations available as a string in a textfield just like in a google-search. When this is sent the system would parse the expression, check it for sanity, and generate a plan on how to do the calculation on the machine. It would have to take into account things like the order of operations. It would check operand size, (done, see below) reprimand users who ask too much and forward them to Wolfram Alpha. It may even make optimizations to utilize shortcuts as described in the manual. Then, when the machine becomes available, the robotic arm would do the calculation on it, operating the levers and cranks just like a human would. The user could view progress through a webcam and read off the final result. As a side-effect the process could be logged step-by-step to explain how the general expression is translated onto the machine.
I love to imagine the sight of a pretty industrial robot or a shitty homebuilt one going through the motions. Oh, and the delicious noises both obsolete and modern machine would make!
That's cool, why doesn't it exist?
You ask, and right you are.
82% of the answer is that I am a software person.
My understanding of physical robots is limited to playing with a LEGO Mindstorms set and a few servos connected to an Arduino and nothing else (so all they did was make some noise and wiggle themselves across the table).
78% of the answer is that I am chicken and lazy.
My main concern is that I am not really able to select a suitable arm. Be it ready-made, in kit-form, as plans or even design my own. I don't have the know-how to think through how many and what axes with what reach would be required to elegantly access all the controls on the machine.
And I have no idea what the requirements on repeatability and payload are or how to measure them. The machine turns quite easily, but the hardest to pull is the reset-all-handle and I estimate that to be about 2kg (using rubber bands, a tape measure and a kitchen scale as tools).
And of course anything I actually embark on would have to remain reasonably priced. Whatever "reasonable" means in the context of such a ludicrous project.
Help wanted
So.
Do you have input to give? Are you knowledgeable in the subjects I am ignorant of?
Do you want to contribute?
Do you have hardware lying around looking for a purpose in life?
Have you seen or done something similar? Do you want to encourage me or rather tell me why the idea is dumb – other than the obvious uselessness of it?
If your answer to any of the questions above is yes, please contact me. I am happy to chat about this in any level of detail and also open to all and any arrangement that may take my vision closer to reality.
The Brunsviga-Assembler
Software is easy (to me), so I started on that. I looked at the Brunsviga as if it was part of a modern computer. It can do some arithmetic so it is an ALU and has Registers. It does not have control-flow, however. That is provided by the operator. Traditionally a human, but that does not have to remain like that!
I then designed an instruction set for it and implemented an assembler in Python. The assembler is given an arithmetic expression in string form and outputs an instruction stream that performs the calculation on the machine.
I have since ported that piece of code to JavaScript and an implementation can be run in your browser in a (very) simple webpage from here.
Both implementations use third-party parsers for the expression, so don't go looking for that in the code. The idiosyncrasies of said parsers drive most of the differences between the Python and JavaScript versions (also the JS-Version has considerably out-evolved the one in Python by now). One notable example is that math.js insists on using unary minus instead of just putting a negative number as constant node. The code replaces those instances with an explicit subtraction from zero which yields the exact same result without any special coding for the calculator.
Machine model
The model used to describe the Brunsviga is an accumulator machine with the Resultatwerk as the Accumulator, called R-Register. There is one Register for an operand, the Einstellwerk E. And one register similar to a status-register, the Umdrehungszählwerk U. The instructions for the machine also refer to the Anzeigewerk, but that is simply the numeric display and parallels the Einstellwerk E.
For this machine I have defined an instruction set and a notation to write this down as a sort of Assembler-program. Each instruction encapsulates an action on the machine. The instructions set is designed to keep programs in it just readable.
To make a robot execute it the instructions will have to broken down a little bit further, very much like microcode or instruction sequencing in an electronic CPU. But I expect that to be very straightforward. It can most propably be done just by recalling positions from teach-in memory.
In addition to instructions the assember-language also defines comments. Anything after and including a semicolon is ignored. The assembler generates comments as part of it's execution so a human can follow the "code" more easily.
Note that there are no instructions for control-flow. This is a symptom of the ALU-ness of the machine model.
Going from AST to the program for the Brunsviga is very straightforward. The code includes a few very simple local optimizations a human operator could apply without modifying the problem. These include:
- Not loading a value if the same is already in the register. It is assumed a person could see this and skip the step.
- Swapping the operands for a multiplication so the one with less figures is the second operand.
- If one of the operands of an addition or multiplication (commutative operations) needs some computation itself that is done first so the result will already be in the R-Werk and does not require a separate step to be loaded.
RESETR
The RESETR instructions resets the Resultatwerk R to zero.
LOAD n
The LOAD instruction takes an integer operand n and sets the value in the Einstellwerk E. The value is automatically displayed in the Anzeigewerk A.
CRANKFWD n
Turn the Crank forward n times. Effectively adds the value in the Einstellwerk E to the Resultatwerk R with proper scaling.
CRANKREV n
Turn the Crank backwards n times. Effectively substracts the value in the Einstellwerk E from the Resultatwerk R with proper scaling. If there is an underflow the bell sounds.
SCALEUP n
Shift the scale up n steps for a factor of 10^n.
SCALEDWN n
Shift the scale down n steps by a factor of 10^-n.
DONE
DONE is not a a real instruction. It signifies the end of the program and does not change machine state.
Examples
; 1 + 2 + 3
LOAD 1
CRANKFWD 1
LOAD 2
CRANKFWD 1
LOAD 3
CRANKFWD 1
DONE
; 12 * 13
LOAD 13
CRANKFWD 2
SCALEUP
CRANKFWD 1
SCALEDWN
DONE
;(12 + 45) * 607
LOAD 12
CRANKFWD 1
LOAD 45
CRANKFWD 1
LOAD 607
RESETR
RESETU
CRANKFWD 7
SCALEUP
CRANKFWD 5
SCALEDWN
DONE